How to Log Messages and Debug in CloudPages: Server Side Javascript Examples
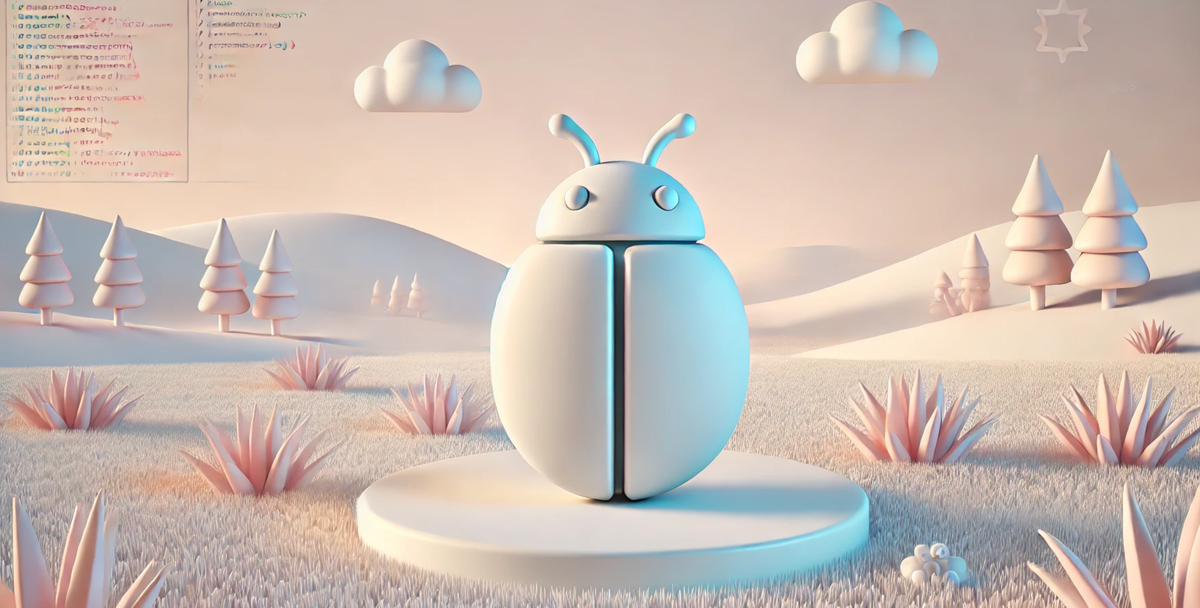
Developing in Salesforce Marketing Cloud Web Studio can sometimes be frustrating, especially if you're using server-side Javascript or AMPscript to perform actions on your page.
In this post, I'll go through my approach to log messages and debug in CloudPages. Here's what we will cover:
- What causes 500 errors in CloudPages?
- How to catch 500 errors in CloudPages using Serverside Javascript
- Take logging and debugging in CloudPages to the next level using a global variable
Let's start!
What causes 500 errors in CloudPages?
t doesn't matter if you are an experienced developer or someone that is just starting with CloudPages; you have probably seen this page many times:

500 - Internal Server error is a very generic code and message web servers show whenever something goes wrong. n Marketing Cloud, this is generally caused by situations such as:
- Syntax error in server-side Javascript (like when you misspell a function name or write
lenght
instead oflength
). - Logical errors in SSJS or AMPScript (such as calling a variable that is not defined or passing the wrong attribute to a function).
... and many other causes.
Since CloudPages does not have a debugging mode (it has been an idea for years), it is up to the developer to deal with those errors. And that can be very intimidating, especially if you are a beginner or have no background in programming.
How to catch most 500 errors in CloudPages using Server-side Javascript?
Server Side Javascript (SSJS) can help with debugging and make developing for CloudPages a much more enjoyable experience. But only if you take the time to configure a few things first. Let's start with the basics: a try-catch block.
A try-catch block is essentially a way totry
to run some code and, if that code fails,catch
the error and deal with it instead of ruining our whole page with a 500 error.
Let's start with the basic syntax for a try-catch statement in SSJS.
<script runat="server">
Platform.Load("core", "1.1.1")
try{
//We will try executing code here. And if it fails..
}catch(e){
// we catch the error in a variable called "e" and deal with it before we ..
}finally{
// optionally run some code regardless of success or failure.
}
</script>
Basic SSJS Code Try-catch statement
You can also use a try-catch block with AMPscript. Close your script
tag after you open the try
statement, write the AMPScript, and add a second script
tag where you close the try
and do the catch
.<script runat="server">
Platform.Load("core", "1.1.1")
try{
</script>
%%[
/* Your AMPScript code goes here. If things go wrong here.. */
]%%
<script runat="server">
}catch(e){
// we catch the error in a variable called "e" and deal with it before we ..
}finally{
// optionally run some code regardless of success or failure.
}
</script>
SSJS try-catch statement with AMPScript
What can we do inside the catch
block? We can display the error on the page, record it in a data extension, redirect the user to an error page or ignore it (but don't ignore it. Seriously).
Here is how you can display the error on the page, which can be handy while debugging:
//...
try{
//... the rest of your code that threw the error
}catch(e){
//print the error on the Screen
Write(Stringify(e))
}finally{
//...
}
SSJS Try-Catch statement with Write
While the above can catch many errors, 500 server errors can still occur and are usually caused by syntax errors. One approach to locating what is causing the error is to comment out your code in sections starting at the bottom until the error goes away. Sometimes it's just an extra comma hiding somewhere.
Take logging and debugging in CloudPages to the next level using a global variable and a custom log function
After you finish testing or debugging a CloudPage, you can end up with dozens of Write('..')
on your code. Now you have to go through all of the lines of code and remove them before publishing.
Need to test things again? Add all the Write('..')
statements back.. Need to publish the page? Remove them... Need to test? ...you get the idea.
Using a custom log
function and a global SETTINGS
variable (you can name them anything) lets you turn logging on and off very easily. Let's see how to do that. Here is what my SETTINGS
variable and log
function usually look like:
// setting DEBUG to false urns off all messages
var SETTINGS = {
"DEBUG": true
}
//function to log messages on the screen
function log(message){
if(SETTINGS["DEBUG"]){
//if we pass an object, we first use Stringify before printing
if(typeof(message) == "object"){
Write(Stringify(message) + "<br><br>") // we also print a couple of line breaks
}else{
Write(message + "<br><br>")
}
}
}
try{
// here is my code and I'm about to log something on the screen
log("Hello World, I only display if debug is true")
//...
}catch(e){
//...
}
By adding this to the very top of my page and using log()
instead of Write()
, you can set the debug setting to false
, and all the messages won't display on the page. You can also add logic to save the errors in a data extension when debugging is off, or maybe send yourself a triggered email with the error.
Bonus tip: You can even take that a step further and create a custom Redirect function to stop the page from sending the user to another URL, such as success and error pages. It can be very valuable while debugging forms that redirect the user after submission is complete:
function customRedirect(url){
// If debug is on, we only print a message on the page instead
// of redirecting the user
if(SETTINGS["DEBUG"]){
log("Redirect User to " + url)
}else{
Redirect(URL)
}
}
customRedirect("www.mysuccess-page-url.com")
// the function would print "Redirect User to to www.mysuccess-page-url.com"
I hope the content above was useful and you have learned something new. What was your most frustarting error that ended up being simple to fix? Mine was definitely misspelling the word length
.
I'll be posting more content like this, so check back for future posts. Leave suggestions in the comments for topics you'd like to learn more about in SFMC.
Happy coding!